This is a quick and easy way to send a bot message on Discord using Python, without the discord.py library, by simply doing a POST request with the requests library.
First you’ll need your bot’s token, which you can find from: https://discord.com/developers/applications
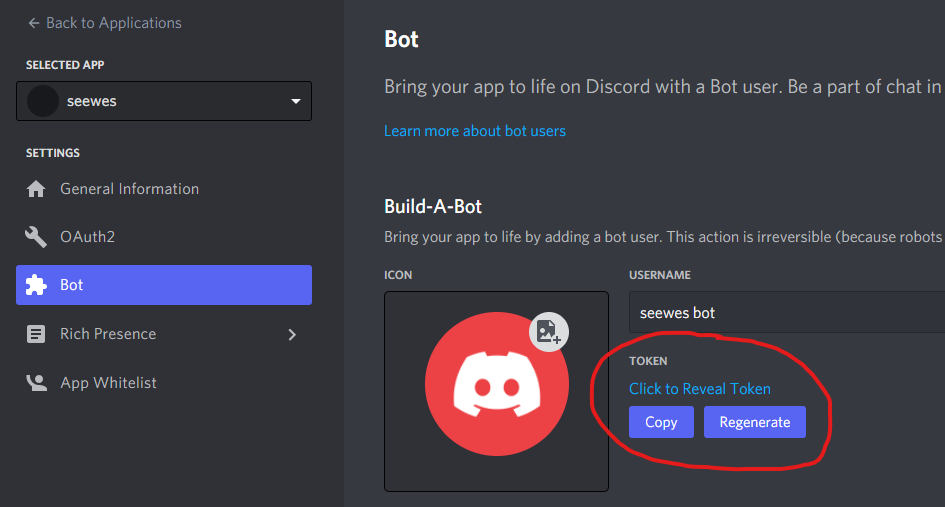
Next you’ll need the ID of the text channel where you want to send the messages to be sent. Simply right click the channel name and click “Copy ID”.
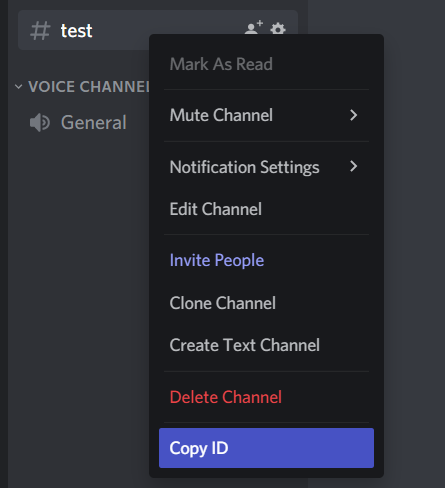
With those 2 values copied, paste them into the respective variables in the code below.
import requests
BOT_TOKEN = 'xxxxx'
CHANNEL_ID = '#####'
message = 'hello world!'
requests.post(
url='https://discord.com/api/v8/channels/{}/messages'.format(CHANNEL_ID),
json={'content': message},
headers={
'authorization': 'Bot {}'.format(BOT_TOKEN),
'content-type': 'application/json',
}
)
And that’s it! Execute the python script and check the Discord channel for the message!
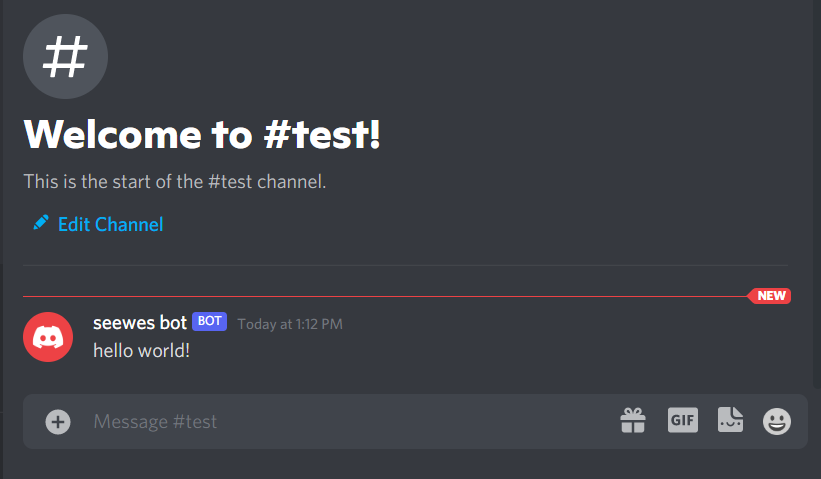